1) How we lock/unlock a EditText in android ?
or
How we prevent EditText to take input in android ?
Answer :- Here is the sample project to show how we can prevent "EditText" to take input in android.
main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="fill_parent"
android:layout_height="fill_parent">
<EditText android:id="@+id/textbox" android:layout_width="fill_parent"
android:layout_height="wrap_content"/>
<Button android:id="@+id/btnLockUnlock" android:layout_width="wrap_content" android:layout_height="wrap_content"/>
</LinearLayout>
Main.java
package com.androd.satya;
import android.os.Bundle;
import android.text.InputFilter;
import android.text.Spanned;
import android.view.View;
import android.app.Activity;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class Main extends Activity{
private EditText editText;
private boolean value = false;
@Override
public void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
editText = (EditText) findViewById(R.id.textbox);
Button btnLockUnlock = (Button) findViewById(R.id.btnLockUnlock);
btnLockUnlock.setText("Lock/Unlock");
btnLockUnlock.setOnClickListener(new View.OnClickListener(){
@Override
public void onClick(View v){
if (value){
value = false;
} else{
value = true;
}
lockUnlock(value);
}
});
}
private void lockUnlock(boolean value){
if (value){
Toast.makeText(this, "Lock the EditText", Toast.LENGTH_LONG).show();
editText.setFilters(new InputFilter[]{
new InputFilter(){
@Override
public CharSequence filter(CharSequence source,int start,int end,Spanned dest,int dstart,int dend) {
return source.length() < 1 ? dest.subSequence(dstart,dend):"";
}
}
});
} else{
Toast.makeText(this, "UnLock the EditText", Toast.LENGTH_LONG).show();
editText.setFilters(new InputFilter[]{
new InputFilter(){
//@Override
public CharSequence filter(CharSequence source,int start,int end,Spanned dest,int dstart,int dend){
return null;
}
}
});
}
}
}
Monday, October 24, 2011
Tuesday, July 19, 2011
Changing the battery percentage in an AVD (android virtual device) emulator
Question :- How we change the battery percentage in an AVD (android virtual device) Emulator ?
Answer :- Open an Emulator : 5554
Go to "C:\Program Files\Android\android-sdk\platform-tools>" and type a command : "telnet localhost 5554".
After then in same window telnet client starts. Type "help" command there.
"help" command gives you the list of all commands used by emulator. Type "help power" command there.
"help power" command gives you the list of all sub-commands used by "power" command. Type "power capacity 80" command there.
This will change your battery percentage of emulator. You can also give command like :
power capacity 40
power capacity 70
power capacity 10
power capacity 50
Battery percentage is reflected only on Emulator.
Tuesday, July 5, 2011
How we check Battery Percentage in Android
Make a new project in android.
import android.app.Activity;
In BatteryLevelActivity.java
package com.android.checkbatterylevel;
import android.content.Intent;
import android.content.IntentFilter;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
public class BatteryLevelActivity extends Activity {
/** Called when the activity is first created. */
public static TextView txt;
Button btn;
BatteryChangeReceiver batteryReceiver = null;
IntentFilter batFilter = null;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
txt = (TextView)findViewById(R.id.text1);
btn = (Button)findViewById(R.id.button1);
btn.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) { // TODO Auto-generated method stub
batteryReceiver = new BatteryChangeReceiver();
batFilter = new IntentFilter(Intent.ACTION_BATTERY_CHANGED);
registerReceiver(batteryReceiver, batFilter);
}
});
}
}
In BatteryChangeReceiver.java
package com.android.checkbatterylevel;
import android.content.BroadcastReceiver;
import android.content.Context;
import android.content.Intent;
import android.util.Log;
public class BatteryChangeReceiver extends BroadcastReceiver{
public final String ACTION1 = Intent.ACTION_BATTERY_CHANGED;
@Override
public void onReceive(Context arg0, Intent intent) {
if(intent.getAction().equals(ACTION1)){
int level = intent.getIntExtra("level", 0);
BatteryLevelActivity.txt.setText("Battery Level is-> " + level);
Log.e("Battery Level : "," : "+level);
}
}
}
In main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:id="@+id/text1"/>
<Button android:text="Check Battery Level"
android:id="@+id/button1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</LinearLayout>
In AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.android.checkbatterylevel"
android:versionCode="1"
android:versionName="1.0">
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".BatteryLevelActivity"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<receiver android:name="BatteryChangeReceiver"></receiver>
</application>
</manifest>
Run the program you will get like this :
Tuesday, June 21, 2011
Release Memory, Valid URL, Valid Email, Valid Phone
1) How we release a memory in android ?
Answer :- Suppose if we use in our activity
Button btnYes;
LinearLayout llMain;
Gallery gallery
ImageButton btnSearch
ImageView backImageView;
Answer :- Suppose if we use in our activity
Button btnYes;
LinearLayout llMain;
Gallery gallery
ImageButton btnSearch
ImageView backImageView;
@Override
protected void onPause() {
releaseViewMemory(btnYes);
releaseViewMemory(llMain);
releaseViewMemory(gallery);
releaseViewMemory(btnSearch);
releaseImageViewMemory(backImageView);
super.onPause();
}
public static void releaseViewMemory(View view) {
if (view != null) {
Drawable draw = view.getBackground();
if (draw != null) {
draw.setCallback(null);
draw = null;
}
}
}
public static void releaseImageViewMemory(ImageView imgView) {
if (imgView != null) {
Drawable draw = imgView.getBackground();
if (draw != null) {
draw.setCallback(null);
draw = null;
}
draw = imgView.getDrawable();
if (draw != null) {
draw.setCallback(null);
draw = null;
}
}
}
2) Write a method for valid url.
Answer :-
protected void onPause() {
releaseViewMemory(btnYes);
releaseViewMemory(llMain);
releaseViewMemory(gallery);
releaseViewMemory(btnSearch);
releaseImageViewMemory(backImageView);
super.onPause();
}
public static void releaseViewMemory(View view) {
if (view != null) {
Drawable draw = view.getBackground();
if (draw != null) {
draw.setCallback(null);
draw = null;
}
}
}
public static void releaseImageViewMemory(ImageView imgView) {
if (imgView != null) {
Drawable draw = imgView.getBackground();
if (draw != null) {
draw.setCallback(null);
draw = null;
}
draw = imgView.getDrawable();
if (draw != null) {
draw.setCallback(null);
draw = null;
}
}
}
2) Write a method for valid url.
Answer :-
public static boolean isValidURL(String url) {
boolean isValid = false;
String expression = "^(http|https|ftp)\\://[a-zA-Z0-9\\-\\.]+\\.[a-zA-Z]{2,3}(:[a-zA-Z0-9]*)?/?([a-zA-Z0-9\\-\\._\\?\\,\\'/\\\\+&%\\$#\\=~])*$";
CharSequence inputStr = url;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputStr);
if (matcher.matches()) {
isValid = true;
}
return isValid;
}
3) Write a method for valid email.
Answer :-
String expression = "^(http|https|ftp)\\://[a-zA-Z0-9\\-\\.]+\\.[a-zA-Z]{2,3}(:[a-zA-Z0-9]*)?/?([a-zA-Z0-9\\-\\._\\?\\,\\'/\\\\+&%\\$#\\=~])*$";
CharSequence inputStr = url;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputStr);
if (matcher.matches()) {
isValid = true;
}
return isValid;
}
3) Write a method for valid email.
Answer :-
public static boolean isValidEmail(String email) {
boolean isValid = false;
String expression = "^[\\w\\.-]+@([\\w\\-]+\\.)+[A-Z]{2,4}quot;;
CharSequence inputStr = email;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputStr);
if (matcher.matches()) {
isValid = true;
}
return isValid;
}
3) Write a method for valid phone.
Answer :-
public static boolean isValidphone(String phone) {
boolean isValid = false;
String expression = "^[+][0-9]{10,13}$";
CharSequence inputstr = phone;
if (phone.length() == 0)
return false;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputstr);
if (matcher.matches()) {
if (phone.length() == 10)
isValid = true;
}
return isValid;
}
boolean isValid = false;
String expression = "^[\\w\\.-]+@([\\w\\-]+\\.)+[A-Z]{2,4}quot;;
CharSequence inputStr = email;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputStr);
if (matcher.matches()) {
isValid = true;
}
return isValid;
}
3) Write a method for valid phone.
Answer :-
public static boolean isValidphone(String phone) {
boolean isValid = false;
String expression = "^[+][0-9]{10,13}$";
CharSequence inputstr = phone;
if (phone.length() == 0)
return false;
Pattern pattern = Pattern.compile(expression, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(inputstr);
if (matcher.matches()) {
if (phone.length() == 10)
isValid = true;
}
return isValid;
}
Thursday, June 2, 2011
Core Java Questions Part-2
1) What is Collection ?
Answer : Collection — the root of the collection hierarchy. A collection represents a group of objects known as its elements. The Java platform doesn't provide any direct implementations of this interface but provides implementations of more specific subinterfaces, such as Set and List.
è Set — A Set is a Collection that cannot contain duplicate elements. It models the mathematical set abstraction. The Set interface contains only methods inherited from Collection and adds the restriction that duplicate elements are prohibited.
Ø SortedSet — a Set that maintains its elements in ascending order.
è List — A List is an ordered Collection (sometimes called a sequence). Lists may contain duplicate elements.
Ø ArrayList - Resizable-array implementation of the List interface. Implements all optional list operations, and permits all elements, including null.
Ø LinkedList
Ø Vector - The Vector class implements a growable array of objects. Like an array, it contains components that can be accessed using an integer index.
è Queue — A collection used to hold multiple elements prior to processing. Besides basic Collection operations, a Queue provides additional insertion, extraction, and inspection operations.
è Map — An object that maps keys to values. A Map cannot contain duplicate keys; each key can map to at most one value.
o SortedMap — A Map that maintains its mappings in ascending key order.
2) What is the difference between List, Set and Map?
Answer : A Set is a collection that has no duplicate elements. A List is a collection that has an order associated with its elements. A map is a way of storing key/value pairs. The way of storing a Map is similar to two-column table.
3) What is the difference between Vector and ArrayList ?
Answer : Vector is synchronized, ArrayList is not. Vector is having a constructor to specify the incremental capacity. But ArrayList don't have. By default Vector grows by 100% but ArrayList grows by 50% only.
4) What is the difference between Hashtable and HashMap ?
Answer : Hashtable is synchronized . but HashMap is not synchronized. Hashtable does not allow null values , but HashMap allows null values.
5) What is the difference between array and ArrayList ?
Answer : Array is collection of same data type. Array size is fixed, It cannot be expanded. But ArrayList is a growable collection of objects. ArrayList is a part of Collections Framework and can work with only objects.
6) What is any an anonymous class?
Answer : An anonymous class is a local class with no name.
7) What is the difference between synchronized block and synchronized method ?
3) What is the difference between Vector and ArrayList ?
Answer : Vector is synchronized, ArrayList is not. Vector is having a constructor to specify the incremental capacity. But ArrayList don't have. By default Vector grows by 100% but ArrayList grows by 50% only.
4) What is the difference between Hashtable and HashMap ?
Answer : Hashtable is synchronized . but HashMap is not synchronized. Hashtable does not allow null values , but HashMap allows null values.
5) What is the difference between array and ArrayList ?
Answer : Array is collection of same data type. Array size is fixed, It cannot be expanded. But ArrayList is a growable collection of objects. ArrayList is a part of Collections Framework and can work with only objects.
6) What is any an anonymous class?
Answer : An anonymous class is a local class with no name.
7) What is the difference between synchronized block and synchronized method ?
Answer : Synchronized blocks place locks for the specified block where as synchronized methods place locks for the entire method.
Wednesday, May 25, 2011
Core Java Questions Part-1
Why Java is not 100% pure object oriented language?
Answer : Because java uses primitives.
What is the difference between static and non static variables ?
Answer : A static variable is associated with the class as a whole rather than with specific instances of a class. There will be only one value for static variable for all instances of that class. Non-static variables take on unique values with each object instance
What is static initializer block? What is its use?
Answer : A static initializer block is a block of code that declares with the static keyword. It normally contains the block of code that must execute at the time of class loading. The static initializer block will execute only once at the time of loading the class only
What are the methods in Object?
Answer : clone, equals, wait, finalize, getClass, hashCode, notify, notifyAll, toString
What is daemon thread?
Answer : Theards which are running on the background are called deamon threads. daemon thread is a thread which doesn't give any chance to run other threads once it enters into the run state it doesn't give any chance to run other threads. Normally it will run forever, but when all other non-daemon threads are dead, daemon thread will be killed by JVM
What is Transient variables ?
Answer : Transient variables are variable that cannot be serialized.
What is synchronization
Answer : Synchronization is the ability to control the access of multiple threads to shared resources. With synchronization , at a time only one thread will be able to access a shared resource.
What are the differences between an abstract class and an interface?
Answer : An abstract class can have concrete method, which is not allowed in an interface. Abstract class can have private or protected methods and variables and only public methods and variables are allowed in interface. We can implement more than one interface , but we can extend only one abstract class. Interfaces provides loose coupling where as abstract class provides tight coupling.
What are different type of exceptions in Java?
Answer : There are two types of exceptions in java. Checked exceptions and Unchecked exceptions. Any exception that is is derived from Throwable and Exception is called checked exception except RuntimeException and its sub classes. The compiler will check whether the exception is caught or not at compile time. We need to catch the checked exception or declare in the throws clause. Any exception that is derived from Error and RuntimeException is called unchecked exception. We don't need to explicitly catch a unchecked exception.
What is an abstract class and Abstract Method?
Answer : An abstract class is an incomplete class. It is declared with the modifier abstract. We cannot create objects of the abstract class. It is used to specify a common behavioral protocol for all its child classes.
An abstract method is a method that don't have a body. It is declared with modifier abstract.
What is an interface?
Answer : An interface is a collection of method declarations and constants. In java interfaces are used to achieve multiple inheritance. It sets a behavioral protocol to all implementing classes.
What is finalize() ?
Answer : Finalize is a protected method in java. When the garbage collector is executes , it will first call finalize( ), and on the next garbage-collection it reclaim the objects memory. So finalize( ), gives you the chance to perform some cleanup operation at the time of garbage collection.
What is final ?
Answer : A final is a keyword in java. If final keyword is applied to a variable, then the variable will become a constant. If it applied to method, sub classes cannot override the method. If final keyword is applied to a class we cannot extend from that class.
What is static ?
Answer : static means one per class. static variables are created when the class loads. They are associated with the object. In order to access a static we don't need objects. We can directly access static methods and variable by calling classname.variablename.
How we remove white spaces from a string ?
Answer :- Use trim() method to remove a white spaces.
String str = " Satya";
str.trim();
Friday, April 15, 2011
SDCARD
1) How we setup sdcard and copy data into sdcard in emulator ?
Answer :-
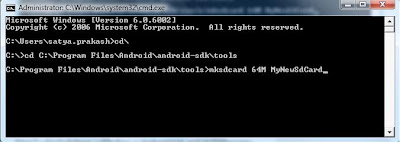
Step 2 :- Go to Eclipse-> Window -> Android SDK and AVD Manager
Step 3:- Select a emulator in which you want to add sdcard and click on “Edit”

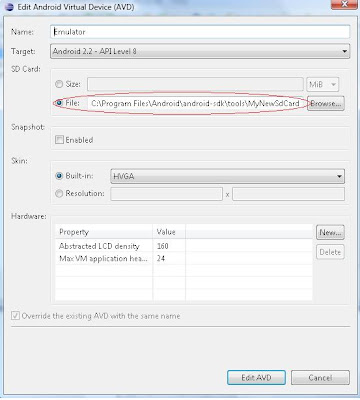
Step 5 :- Press Edit AVD.
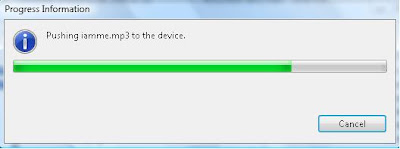
Answer :-
Setup sdcard :-
Step 1 :- Open a command promt. Go to android-sdk\tools and run following command there.
mksdcard 64M MyNewSdCard
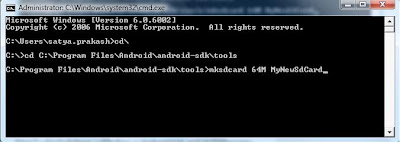
Step 2 :- Go to Eclipse-> Window -> Android SDK and AVD Manager
Step 3:- Select a emulator in which you want to add sdcard and click on “Edit”

Step 4 :- Select the “File” option button from SD Card. Give the path where you save the sdcard “MyNewSdCard”. In my case “ C:\Program Files\Android\android-sdk\tools\MyNewSdCard ”
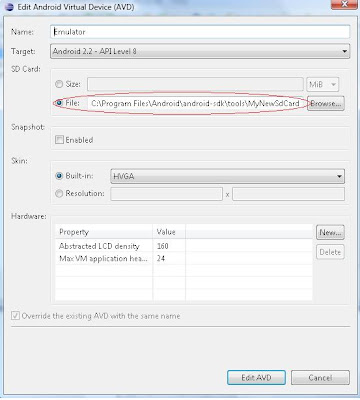
Step 5 :- Press Edit AVD.
- Push the file on sdcard. Here I puch iamme.mp3 on sdcard.
Step 2 :- Run ddms.bat from “C:\Program Files\Android\android-sdk\tools\ddms.bat”.
Step 3 :- Select the Emulator and after then press on Device-> File Explorer
Step 4 :- In File Explorer select sdcard then select “Push file onto Device”
It will show a progress dialog box like
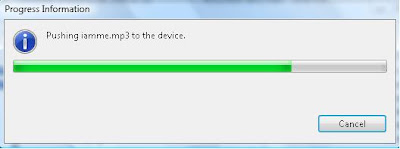
After then it will show like this.
Tuesday, March 29, 2011
Localization, Boot Process and Sensor
1) What is localization and how to achieve it ?
Answer :- Android will run on many devices in many regions. To reach the most users, your application should handle text, audio files, numbers, currency, and graphics in ways appropriate to the locales where your application will be used. For more details you can refer : http://developer.android.com/resources/tutorials/localization/index.html
3) What are the launch mode in android? What are the types of launch mode for an activity and which one is default ?
Answer :- An instruction on how the activity should be launched. There are four modes that work in conjunction with activity flags (FLAG_ACTIVITY_* constants) inIntent objects to determine what should happen when the activity is called upon to handle an intent. They are:
"standard"
"singleTop"
"singleTask"
"singleInstance"
The default mode is "standard".
<activity>
android:launchMode=["multiple" | "singleTop" | "singleTask" | "singleInstance"]
</activity>
4) Give a example of implicit intent and explicit intent ?
Answer :-
Implicit Intents - Opening an URL
Intent implicitIntent = new Intent();
Uri url=Uri.parse("http://www.ea.com");
implicitIntent.setAction(Intent.ACTION_VIEW);
implicitIntent.setData(url);
context.startActivity(implicitIntent);
Explicit intents - Data transfer between activities
Intent explicitIntent = new Intent( this, anotherActivity.class );
explicitIntent.putExtra( "int", 5);
explicitIntent.putExtra( "string", "hello" );
startActivity(explicitIntenti);
Answer :- Android will run on many devices in many regions. To reach the most users, your application should handle text, audio files, numbers, currency, and graphics in ways appropriate to the locales where your application will be used. For more details you can refer : http://developer.android.com/resources/tutorials/localization/index.html
2) You have 4 activity in your application Activity1, Activity2, Activity3, Activity4 and i want to make one of them as the main activity so that when the user select the application in the launcher that particular activity is shown how you do this ?
Answer :- Suppose if i want to make Activity3 as the main activity then we have to make a changes in AndroidManifest.xml .
<activity class=".Activity3" android:label="@string/Activity_3">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
Answer :- An instruction on how the activity should be launched. There are four modes that work in conjunction with activity flags (FLAG_ACTIVITY_* constants) inIntent objects to determine what should happen when the activity is called upon to handle an intent. They are:
"standard"
"singleTop"
"singleTask"
"singleInstance"
The default mode is "standard".
<activity>
android:launchMode=["multiple" | "singleTop" | "singleTask" | "singleInstance"]
</activity>
Answer :-
Implicit Intents - Opening an URL
Intent implicitIntent = new Intent();
Uri url=Uri.parse("http://www.ea.com");
implicitIntent.setAction(Intent.ACTION_VIEW);
implicitIntent.setData(url);
context.startActivity(implicitIntent);
Explicit intents - Data transfer between activities
Intent explicitIntent = new Intent( this, anotherActivity.class );
explicitIntent.putExtra( "int", 5);
explicitIntent.putExtra( "string", "hello" );
startActivity(explicitIntenti);
5) What is the android boot process from power on ?
Answer :- I don't have too much experience in android to give the answer of this question, so please visit this url. This is helpfull to understand the boot process of android devices from power on. http://www.androidenea.com/2009/06/android-boot-process-from-power-on.html
6) What is Sensor in Android ?
Answer :- SensorManager lets you access the device's sensors. Get an instance of this class by calling Context.getSystemService() with the argumentSENSOR_SERVICE. Always make sure to disable sensors you don't need, especially when your activity is paused. Failing to do so can drain the battery in just a few hours. Note that the system will not disable sensors automatically when the screen turns off.
public class SensorActivity extends Activity, implements SensorEventListener {
private final SensorManager mSensorManager;
private final Sensor mAccelerometer;
public SensorActivity() {
mSensorManager =
6) What is Sensor in Android ?
Answer :- SensorManager lets you access the device's sensors. Get an instance of this class by calling Context.getSystemService() with the argumentSENSOR_SERVICE. Always make sure to disable sensors you don't need, especially when your activity is paused. Failing to do so can drain the battery in just a few hours. Note that the system will not disable sensors automatically when the screen turns off.
public class SensorActivity extends Activity, implements SensorEventListener {
private final SensorManager mSensorManager;
private final Sensor mAccelerometer;
public SensorActivity() {
mSensorManager =
(SensorManager)getSystemService(SENSOR_SERVICE);
mAccelerometer =
mAccelerometer =
mSensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
}
protected void onResume() {
super.onResume();
mSensorManager.registerListener(this, mAccelerometer,
}
protected void onResume() {
super.onResume();
mSensorManager.registerListener(this, mAccelerometer,
SensorManager.SENSOR_DELAY_NORMAL);
}
protected void onPause() {
super.onPause();
mSensorManager.unregisterListener(this);
}
public void onAccuracyChanged(Sensor sensor, int accuracy) {
}
public void onSensorChanged(SensorEvent event) {
}
}
protected void onPause() {
super.onPause();
mSensorManager.unregisterListener(this);
}
public void onAccuracyChanged(Sensor sensor, int accuracy) {
}
public void onSensorChanged(SensorEvent event) {
}
}
7) What is data synchronization in android ?
7) What is data synchronization in android ?
Answer :-
8) Suppose i have one Activity in application 2 and i want to use that Activity in application 1. How we achieve this ?
Answer :-
Thursday, March 24, 2011
Event, AIDL, Screen Resolution and Orientation
1) How many types of Events in Android ?
Answer :-
onKeyDown :- onKeyDown Called when any device key is pressed; includes the D-pad, keyboard, hang-up, call, back, and camera buttons.
@Override
public boolean onKeyDown(int keyCode, KeyEvent keyEvent) {
// Return true if the event was handled.
return true;
}
onKeyUp :- onKeyUp Called when a user releases a pressed key.
@Override
public boolean onKeyUp(int keyCode, KeyEvent keyEvent) {
// Return true if the event was handled.
return true;
}
onTrackballEvent :- onTrackballEvent Called when the device’s trackball is moved
@Override
public boolean onTrackballEvent(MotionEvent event ) {
// Get the type of action this event represents
int actionPerformed = event.getAction();
// Return true if the event was handled.
return true;
}
onTouchEvent :- onTouchEvent Called when the touch screen is pressed or released, or it detects movement.
@Override
public boolean onTouchEvent(MotionEvent event) {
// Get the type of action this event represents
int actionPerformed = event.getAction();
// Return true if the event was handled.
return true;
}
2) What is Sandboxing ?
Answer :- Each application in Android runs in its own process. An application cannot directly access another application's memory space. This is called application sandboxing.
3) What is AIDL in Android ? How we achieve Interprocess communication (IPC) protocol in Android ?
Answer :-In order to allow cross-application communication, Android provides an implementation of Interprocess Communication (IPC) protocol. IPC protocols tend to get complicated because of all the marshaling/un marshaling of data that is necessary.
To help with this, Android provides Android Interface Definition Language, or AIDL. It is a lightweight implementation of IPC using a syntax that is very familiar to Java developers, and a tool that automates the stub creation.
4) How we handle multiple screen resolution in Android ?
Answer :-
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<supports-screens
android:smallScreens="true"
android:normalScreens="true"
android:largeScreens="true"
android:xlargeScreens="true"
android:anyDensity="true" />
...
</manifest>
The objective of supporting multiple screens is to create an application that can run properly on any display and function properly on any of the generalized screen configurations supported by the platform.
You can easily ensure that your application will display properly on different screens. Here is a quick checklist:
Answer :-
Changing the Screen Orientation Based on the Accelerometer :- If you want to change the screen orientation automatically based on the positioning of the device, you can use the android:screenOrientation attribute in the AndroidManifest.xml file:
To change to portrait mode, use the ActivityInfo.SCREEN_ORIENTATION_PORTRAIT constant:
Besides using the setRequestOrientation() method, you can also use the android:screenOrientation attribute on the <activity> element in AndroidManifest.xml as follows to fix the activity to a certain orientation:
The above example fixes the activity to a certain orientation (landscape in this case) and prevents the activity from being destroyed; that is, the activity will not be destroyed and the onCreate event will not be fired again when the orientation changes.
Answer :-
onKeyDown :- onKeyDown Called when any device key is pressed; includes the D-pad, keyboard, hang-up, call, back, and camera buttons.
@Override
public boolean onKeyDown(int keyCode, KeyEvent keyEvent) {
// Return true if the event was handled.
return true;
}
onKeyUp :- onKeyUp Called when a user releases a pressed key.
@Override
public boolean onKeyUp(int keyCode, KeyEvent keyEvent) {
// Return true if the event was handled.
return true;
}
onTrackballEvent :- onTrackballEvent Called when the device’s trackball is moved
@Override
public boolean onTrackballEvent(MotionEvent event ) {
// Get the type of action this event represents
int actionPerformed = event.getAction();
// Return true if the event was handled.
return true;
}
onTouchEvent :- onTouchEvent Called when the touch screen is pressed or released, or it detects movement.
@Override
public boolean onTouchEvent(MotionEvent event) {
// Get the type of action this event represents
int actionPerformed = event.getAction();
// Return true if the event was handled.
return true;
}
2) What is Sandboxing ?
Answer :- Each application in Android runs in its own process. An application cannot directly access another application's memory space. This is called application sandboxing.
3) What is AIDL in Android ? How we achieve Interprocess communication (IPC) protocol in Android ?
Answer :-In order to allow cross-application communication, Android provides an implementation of Interprocess Communication (IPC) protocol. IPC protocols tend to get complicated because of all the marshaling/un marshaling of data that is necessary.
To help with this, Android provides Android Interface Definition Language, or AIDL. It is a lightweight implementation of IPC using a syntax that is very familiar to Java developers, and a tool that automates the stub creation.
4) How we handle multiple screen resolution in Android ?
Answer :-
<manifest xmlns:android="http://schemas.android.com/apk/res/android">
<supports-screens
android:smallScreens="true"
android:normalScreens="true"
android:largeScreens="true"
android:xlargeScreens="true"
android:anyDensity="true" />
...
</manifest>
The objective of supporting multiple screens is to create an application that can run properly on any display and function properly on any of the generalized screen configurations supported by the platform.
You can easily ensure that your application will display properly on different screens. Here is a quick checklist:
- Use wrap_content, fill_parent, or the dp unit (instead of px), when specifying dimensions in an XML layout file
- Do not use AbsoluteLayout
- Do not use hard coded pixel values in your code
- Use density and/or resolution specific resources
Answer :-
Changing the Screen Orientation Based on the Accelerometer :- If you want to change the screen orientation automatically based on the positioning of the device, you can use the android:screenOrientation attribute in the AndroidManifest.xml file:
<manifest>
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".Orientation"
android:screenOrientation="sensor"
android:label="@string/app_name">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" /> </intent-filter>
</activity>
</application>
</manifest>
The above specifies that the screen orientation for the Orientation activity is based on the sensor (accelerometer) of the device. If you hold the device upright, the screen will be displayed in portrait mode; if you hold it sideways, it will change to landscape mode.
Changing the Screen Orientation Programmatically :- There are times where you need to ensure that your application is displayed only in a certain orientation. For example, suppose you are writing a game that should only be viewed in landscape mode. In this case, you can programmatically force a change in orientation using the setRequestOrientation() method of the Activity class:
The above specifies that the screen orientation for the Orientation activity is based on the sensor (accelerometer) of the device. If you hold the device upright, the screen will be displayed in portrait mode; if you hold it sideways, it will change to landscape mode.
Changing the Screen Orientation Programmatically :- There are times where you need to ensure that your application is displayed only in a certain orientation. For example, suppose you are writing a game that should only be viewed in landscape mode. In this case, you can programmatically force a change in orientation using the setRequestOrientation() method of the Activity class:
import android.app.Activity;
import android.content.pm.ActivityInfo;
import android.os.Bundle;
public class Orientation extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//---change to landscape mode---
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
}
}
To change to portrait mode, use the ActivityInfo.SCREEN_ORIENTATION_PORTRAIT constant:
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
Besides using the setRequestOrientation() method, you can also use the android:screenOrientation attribute on the <activity> element in AndroidManifest.xml as follows to fix the activity to a certain orientation:
<manifest>
<application android:icon="@drawable/icon" android:label="@string/app_name">
<activity android:name=".UIActivity" android:screenOrientation="landscape" android:label="@string/app_name">
<intent-filter> <action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
The above example fixes the activity to a certain orientation (landscape in this case) and prevents the activity from being destroyed; that is, the activity will not be destroyed and the onCreate event will not be fired again when the orientation changes.
Monday, March 21, 2011
Sound, Vibrate and Data Storage etc.
1) How we play a sound in Android
Answer :- We use android.media.MediaPlayer.
Playing from a Raw Resource :- Perhaps the most common thing to want to do is play back media (notably sound) within your own applications. Doing this is easy:
Put the sound (or other media resource) file into the res/raw folder of your project, where the Eclipse plugin (or aapt) will find it and make it into a resource that can be referenced from your R class
Create an instance of MediaPlayer, referencing that resource using MediaPlayer.create, and then call start() on the instance:
MediaPlayer mp = MediaPlayer.create(context, R.raw.sound_file_1);
mp.start();
To stop playback, call stop(). If you wish to later replay the media, then you must reset() and prepare() the MediaPlayer object before calling start() again. (create() calls prepare() the first time.). To pause playback, call pause(). Resume playback from where you paused with start().
Playing from a File or Stream :- You can play back media files from the filesystem or a web URL:
Create an instance of the MediaPlayer using new
Call setDataSource() with a String containing the path (local filesystem or URL) to the file you want to play
First prepare() then start() on the instance:
MediaPlayer mp = new MediaPlayer();
mp.setDataSource(PATH_TO_FILE);
mp.prepare();
mp.start();
2) What is difference between prepare() and prepareAsync()
Answer :- There are two ways (synchronous vs. asynchronous) that the Prepared state can be reached: either a call to prepare() (synchronous) which transfers the object to the Prepared state once the method call returns, or a call to prepareAsync() (asynchronous) which first transfers the object to the Preparing state after the call returns (which occurs almost right way) while the internal player engine continues working on the rest of preparation work until the preparation work completes. When the preparation completes or when prepare() call returns, the internal player engine then calls a user supplied callback method, onPrepared() of the OnPreparedListener interface, if an OnPreparedListener is registered beforehand via setOnPreparedListener(android.media.MediaPlayer.OnPreparedListener).
The main difference is that when we use files then we call prepare() and when we use streams then we call prepareAsync().
3) How we play vibrate in Android
Answer:-
android.os.Vibrator
public class VibrateGame
{
Vibrator v;
public VibrateGame(Context context)
{
v = (Vibrator)context.getSystemService(Context.VIBRATOR_SERVICE);
}
public void vibrateGame(int ms)
{
v.vibrate(ms);
}
}
4) What is SharedPreferences in Android and how we implement it ?
Answer :- The SharedPreferences class provides a general framework that allows you to save and retrieve persistent key-value pairs of primitive data types. You can useSharedPreferences to save any primitive data: booleans, floats, ints, longs, and strings. This data will persist across user sessions (even if your application is killed).
To get a SharedPreferences object for your application, use one of two methods:
getSharedPreferences() - Use this if you need multiple preferences files identified by name, which you specify with the first parameter.
getPreferences() - Use this if you need only one preferences file for your Activity. Because this will be the only preferences file for your Activity, you don't supply a name.
To write values:
1. Call edit() to get a SharedPreferences.Editor.
2. Add values with methods such as putBoolean() and putString().
3. Commit the new values with commit()
To read values, use SharedPreferences methods such as getBoolean() and getString().
// Create Object
SharedPreferences setting = getSharedPreferences("SAVE_FILE", 0);
SharedPreferences.Editor editor = setting.edit();
// For Store The Value
editor.putInt("ypad", canvas.ypad);
editor.putBoolean("up", canvas.up);
editor.commit();
// For Retrieving The Value
canvas.ypad = setting.getInt("ypad", 0);
canvas.up=setting.getBoolean("up", false);
What do u meant 0 and false in above ?
0 and false - Value to return if this preference does not exist. Throws ClassCastException if there is a preference with this name that is not a long
What do u mean by 0 in SharedPreferences setting = getSharedPreferences("SAVE_FILE", 0);
public abstract SharedPreferences getSharedPreferences (String filename, int mode)
There are 3 types of File creation mode:
0 - MODE_PRIVATE - the default mode, where the created file can only be accessed by the calling application
1 - MODE_WORLD_READABLE - allow all other applications to have read access to the created file.
2 - MODE_WORLD_WRITEABLE - allow all other applications to have write access to the created file.
5) How we implement SQLite in Android ?
Answer :- Android provides full support for SQLite databases. Any databases you create will be accessible by name to any class in the application, but not outside the application. The recommended method to create a new SQLite database is to create a subclass of SQLiteOpenHelper and override the onCreate() method, in which you can execute a SQLite command to create tables in the database. For example:
public class DictionaryOpenHelper extends SQLiteOpenHelper
Answer :- We use android.media.MediaPlayer.
Playing from a Raw Resource :- Perhaps the most common thing to want to do is play back media (notably sound) within your own applications. Doing this is easy:
Put the sound (or other media resource) file into the res/raw folder of your project, where the Eclipse plugin (or aapt) will find it and make it into a resource that can be referenced from your R class
Create an instance of MediaPlayer, referencing that resource using MediaPlayer.create, and then call start() on the instance:
MediaPlayer mp = MediaPlayer.create(context, R.raw.sound_file_1);
mp.start();
To stop playback, call stop(). If you wish to later replay the media, then you must reset() and prepare() the MediaPlayer object before calling start() again. (create() calls prepare() the first time.). To pause playback, call pause(). Resume playback from where you paused with start().
Playing from a File or Stream :- You can play back media files from the filesystem or a web URL:
Create an instance of the MediaPlayer using new
Call setDataSource() with a String containing the path (local filesystem or URL) to the file you want to play
First prepare() then start() on the instance:
MediaPlayer mp = new MediaPlayer();
mp.setDataSource(PATH_TO_FILE);
mp.prepare();
mp.start();
2) What is difference between prepare() and prepareAsync()
Answer :- There are two ways (synchronous vs. asynchronous) that the Prepared state can be reached: either a call to prepare() (synchronous) which transfers the object to the Prepared state once the method call returns, or a call to prepareAsync() (asynchronous) which first transfers the object to the Preparing state after the call returns (which occurs almost right way) while the internal player engine continues working on the rest of preparation work until the preparation work completes. When the preparation completes or when prepare() call returns, the internal player engine then calls a user supplied callback method, onPrepared() of the OnPreparedListener interface, if an OnPreparedListener is registered beforehand via setOnPreparedListener(android.media.MediaPlayer.OnPreparedListener).
The main difference is that when we use files then we call prepare() and when we use streams then we call prepareAsync().
3) How we play vibrate in Android
Answer:-
android.os.Vibrator
public class VibrateGame
{
Vibrator v;
public VibrateGame(Context context)
{
v = (Vibrator)context.getSystemService(Context.VIBRATOR_SERVICE);
}
public void vibrateGame(int ms)
{
v.vibrate(ms);
}
}
4) What is SharedPreferences in Android and how we implement it ?
Answer :- The SharedPreferences class provides a general framework that allows you to save and retrieve persistent key-value pairs of primitive data types. You can useSharedPreferences to save any primitive data: booleans, floats, ints, longs, and strings. This data will persist across user sessions (even if your application is killed).
To get a SharedPreferences object for your application, use one of two methods:
getSharedPreferences() - Use this if you need multiple preferences files identified by name, which you specify with the first parameter.
getPreferences() - Use this if you need only one preferences file for your Activity. Because this will be the only preferences file for your Activity, you don't supply a name.
To write values:
1. Call edit() to get a SharedPreferences.Editor.
2. Add values with methods such as putBoolean() and putString().
3. Commit the new values with commit()
To read values, use SharedPreferences methods such as getBoolean() and getString().
// Create Object
SharedPreferences setting = getSharedPreferences("SAVE_FILE", 0);
SharedPreferences.Editor editor = setting.edit();
// For Store The Value
editor.putInt("ypad", canvas.ypad);
editor.putBoolean("up", canvas.up);
editor.commit();
// For Retrieving The Value
canvas.ypad = setting.getInt("ypad", 0);
canvas.up=setting.getBoolean("up", false);
What do u meant 0 and false in above ?
0 and false - Value to return if this preference does not exist. Throws ClassCastException if there is a preference with this name that is not a long
What do u mean by 0 in SharedPreferences setting = getSharedPreferences("SAVE_FILE", 0);
public abstract SharedPreferences getSharedPreferences (String filename, int mode)
There are 3 types of File creation mode:
0 - MODE_PRIVATE - the default mode, where the created file can only be accessed by the calling application
1 - MODE_WORLD_READABLE - allow all other applications to have read access to the created file.
2 - MODE_WORLD_WRITEABLE - allow all other applications to have write access to the created file.
5) How we implement SQLite in Android ?
Answer :- Android provides full support for SQLite databases. Any databases you create will be accessible by name to any class in the application, but not outside the application. The recommended method to create a new SQLite database is to create a subclass of SQLiteOpenHelper and override the onCreate() method, in which you can execute a SQLite command to create tables in the database. For example:
public class DictionaryOpenHelper extends SQLiteOpenHelper
{
private static final int DATABASE_VERSION = 2;
private static final String DICTIONARY_TABLE_NAME = "dictionary";
private static final String DICTIONARY_TABLE_CREATE =
"CREATE TABLE " + DICTIONARY_TABLE_NAME + " (" +
KEY_WORD + " TEXT, " +
KEY_DEFINITION + " TEXT);";
DictionaryOpenHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
private static final int DATABASE_VERSION = 2;
private static final String DICTIONARY_TABLE_NAME = "dictionary";
private static final String DICTIONARY_TABLE_CREATE =
"CREATE TABLE " + DICTIONARY_TABLE_NAME + " (" +
KEY_WORD + " TEXT, " +
KEY_DEFINITION + " TEXT);";
DictionaryOpenHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(DICTIONARY_TABLE_CREATE);
}
}
You can then get an instance of your SQLiteOpenHelper implementation using the constructor you've defined. To write to and read from the database, call getWritableDatabase() and getReadableDatabase(), respectively. These both return a SQLiteDatabase object that represents the database and provides methods for SQLite operations.
Android does not impose any limitations beyond the standard SQLite concepts. We do recommend including an autoincrement value key field that can be used as a unique ID to quickly find a record. This is not required for private data, but if you implement a content provider, you must include a unique ID using the BaseColumns._ID constant.
You can execute SQLite queries using the SQLiteDatabase query() methods, which accept various query parameters, such as the table to query, the projection, selection, columns, grouping, and others. For complex queries, such as those that require column aliases, you should use SQLiteQueryBuilder, which provides several convienent methods for building queries.
Every SQLite query will return a Cursor that points to all the rows found by the query. The Cursor is always the mechanism with which you can navigate results from a database query and read rows and columns.
public void onCreate(SQLiteDatabase db) {
db.execSQL(DICTIONARY_TABLE_CREATE);
}
}
You can then get an instance of your SQLiteOpenHelper implementation using the constructor you've defined. To write to and read from the database, call getWritableDatabase() and getReadableDatabase(), respectively. These both return a SQLiteDatabase object that represents the database and provides methods for SQLite operations.
Android does not impose any limitations beyond the standard SQLite concepts. We do recommend including an autoincrement value key field that can be used as a unique ID to quickly find a record. This is not required for private data, but if you implement a content provider, you must include a unique ID using the BaseColumns._ID constant.
You can execute SQLite queries using the SQLiteDatabase query() methods, which accept various query parameters, such as the table to query, the projection, selection, columns, grouping, and others. For complex queries, such as those that require column aliases, you should use SQLiteQueryBuilder, which provides several convienent methods for building queries.
Every SQLite query will return a Cursor that points to all the rows found by the query. The Cursor is always the mechanism with which you can navigate results from a database query and read rows and columns.
View, Intent, IntentFilters, Layout and Adapter etc.
1) What is View
Answer :- This class represents the basic building block for user interface components. A View occupies a rectangular area on the screen and is responsible for drawing and event handling. View is the base class for widgets, which are used to create interactive UI components (buttons, text fields, etc.).
2) What is Intent ? There are two types of Intent what are those and how they are different with each other ?
Answer:- An intent is an Intent object that holds the content of the message. The three components — activities, services, and broadcast receivers — are activated by asynchronous messages called intents. Intents can be divided into two groups: Explicit Intents and Implicit Intents.
10)How we destroy/finish/kill/exit from application in Android ?
Answer :- Call finish()
Answer :- This class represents the basic building block for user interface components. A View occupies a rectangular area on the screen and is responsible for drawing and event handling. View is the base class for widgets, which are used to create interactive UI components (buttons, text fields, etc.).
2) What is Intent ? There are two types of Intent what are those and how they are different with each other ?
Answer:- An intent is an Intent object that holds the content of the message. The three components — activities, services, and broadcast receivers — are activated by asynchronous messages called intents. Intents can be divided into two groups: Explicit Intents and Implicit Intents.
- Explicit Intents have specified a component (via setComponent(ComponentName) or setClass(Context, Class)), which provides the exact class to be run. Often these will not include any other information, simply being a way for an application to launch various internal activities it has as the user interacts with the application.
- Implicit Intents have not specified a component; instead, they must include enough information for the system to determine which of the available components is best to run for that intent
Answer :- A component's intent filters inform Android of the kinds of intents the component is able to handle or we can say that to inform the system which implicit intents they can handle.
3) What are the different UI Layout ?
Answer :- The standard Layouts are:
Answer :- This class is used to instantiate layout XML file into its corresponding View objects. It is never be used directly -- use getLayoutInflater() or getSystemService(String) to retrieve a standard LayoutInflater instance that is already hooked up to the current context and correctly configured for the device you are running on.
5) What is Adapter in Android ?
Answer :- An Adapter object acts as a bridge between an AdapterView and the underlying data for that view. The Adapter provides access to the data items. The Adapter is also responsible for making a View for each item in the data set.
6) What is AdapterView ?
Answer :- An AdapterView is a view whose children are determined by an Adapter.
7) What is ContentValues and Cursor ?
Answer :- ContentValues class is used to store a set of values that the ContentResolver can process.
Cursor interface provides random read-write access to the result set returned by a database query
8) Content Provider is necessary for SQLite.
Answer :- No, Android provides a way for us to expose even our private data to other applications — with a content provider. A content provider is an optional component that exposes read/write access to your application data, subject to whatever restrictions you want to impose
9) How we draw a bitmap image ?
Bitmap imgBall=null;
BitmapDrawable b=(BitmapDrawable)getResources().getDrawable(R.drawable.ball);
imgBall=b.getBitmap();
canvas.drawBitmap(imgBall, xball, yball, paintObject);
3) What are the different UI Layout ?
Answer :- The standard Layouts are:
- LinearLayout :- A Layout that arranges its children in a single column or a single row. The direction of the row can be set by calling setOrientation().
- RelativeLayout :- A Layout where the positions of the children can be described in relation to each other or to the parent.
- TableLayout :- A layout that arranges its children into rows and columns. The rows are defined in the layout XML, and the columns are determined automatically by Android.
- FrameLayout :- FrameLayout is designed to display a single item at a time. You can have multiple elements within a FrameLayout but each element will be positioned based on the top left of the screen.
- AbsoluteLayout :- A layout that lets you specify exact locations (x/y coordinates) of its children.
Answer :- This class is used to instantiate layout XML file into its corresponding View objects. It is never be used directly -- use getLayoutInflater() or getSystemService(String) to retrieve a standard LayoutInflater instance that is already hooked up to the current context and correctly configured for the device you are running on.
5) What is Adapter in Android ?
Answer :- An Adapter object acts as a bridge between an AdapterView and the underlying data for that view. The Adapter provides access to the data items. The Adapter is also responsible for making a View for each item in the data set.
6) What is AdapterView ?
Answer :- An AdapterView is a view whose children are determined by an Adapter.
7) What is ContentValues and Cursor ?
Answer :- ContentValues class is used to store a set of values that the ContentResolver can process.
Cursor interface provides random read-write access to the result set returned by a database query
8) Content Provider is necessary for SQLite.
Answer :- No, Android provides a way for us to expose even our private data to other applications — with a content provider. A content provider is an optional component that exposes read/write access to your application data, subject to whatever restrictions you want to impose
9) How we draw a bitmap image ?
Bitmap imgBall=null;
BitmapDrawable b=(BitmapDrawable)getResources().getDrawable(R.drawable.ball);
imgBall=b.getBitmap();
canvas.drawBitmap(imgBall, xball, yball, paintObject);
The Paint class holds the style and color information about how to draw geometries, text and bitmaps.
10)How we destroy/finish/kill/exit from application in Android ?
Answer :- Call finish()
Subscribe to:
Posts (Atom)